As I get better at using Tailwind CSS, I can see how much it speeds up my development work. With the huge number of class names, autocomplete is particularly important. However, the official Tailwind VS Code extension has a big limitation: the IntelliSense only appears in certain places.
For example, it works well inside the classname
attribute of a JSX element, but when you want to extract some styles into a string variable or an object for reusability, you lose the autocomplete.
not working
Add custom classRegex
To fix this, we can customize an experimental setting called tailwindCSS.experimental.classRegex
. It lets you specify a list of regular expressions which trigger autocompletion when matched.
Open your settings.json, and the syntax is as follows:
"tailwindCSS.experimental.classRegex": [
"<container with only one classlist>",
["<container regex>", "<class list regex>"]
]
"tailwindCSS.experimental.classRegex": [
"<container with only one classlist>",
["<container regex>", "<class list regex>"]
]
The regex can either be a string or an array, depending on whether you’re matching one or more class lists. Let’s define the terms:
- A classlist is a string containing Tailwind classes. For example,
'bg-black h-full text-sm'
is one class list. - A container contains one or more class lists.
// container with only one class list
tw`bg-black text-sm`
// same, one class list
const a = 'bg-black text-sm'
// container with multiple class list
clsx('bg-black text-sm', 'h-full')
// container with only one class list
tw`bg-black text-sm`
// same, one class list
const a = 'bg-black text-sm'
// container with multiple class list
clsx('bg-black text-sm', 'h-full')
Each regex must contain exactly one capture group, and that’s where the autocomplete pops up.
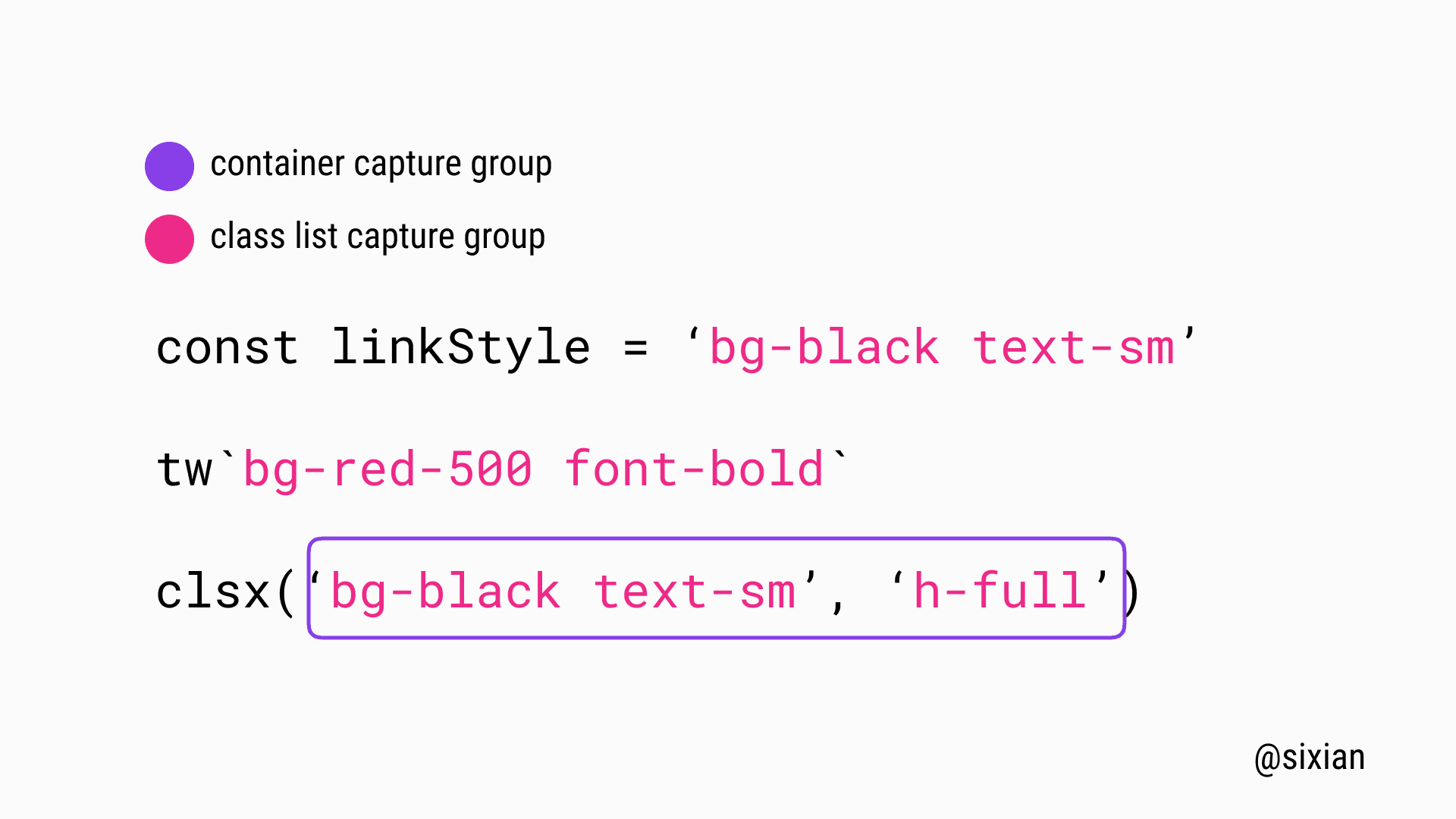
Example1: Variable assignments
Say we want to have autocomplete in variable assignments for variables ending with “Style”, we use [^']
to capture anything inside the single quotes that’s not a single quote.
{
"tailwindCSS.experimental.classRegex": [
// const linkStyle = 'bg-black text-sm'
"Style = '([^']*)'"
]
}
{
"tailwindCSS.experimental.classRegex": [
// const linkStyle = 'bg-black text-sm'
"Style = '([^']*)'"
]
}
To make it more flexible, we can ignore whitespaces and handle double quotes and backticks.
inline object
{
"tailwindCSS.experimental.classRegex": [
// const aStyle = 'text-slate'
// const bStyle = `text-zinc-300`
// const cStyle = "text-red-400"
"Style\\s*=\\s*['\"`]([^'\"`]*)['\"`]"
]
}
{
"tailwindCSS.experimental.classRegex": [
// const aStyle = 'text-slate'
// const bStyle = `text-zinc-300`
// const cStyle = "text-red-400"
"Style\\s*=\\s*['\"`]([^'\"`]*)['\"`]"
]
}
Example2: Object
We have multiple class lists inside an object, so we need the array syntax instead of a single string. The first regex matches anything inside {}
, and the second one matches any string in the values.
{
"tailwindCSS.experimental.classRegex": [
// const styles = { a: '<class lists>', b: '<class lists>'}
["{(.*)}", ": '([^']*)'"]
]
}
{
"tailwindCSS.experimental.classRegex": [
// const styles = { a: '<class lists>', b: '<class lists>'}
["{(.*)}", ": '([^']*)'"]
]
}
To handle multiline objects, we can use [\s\S]
to match everything including newlines. And we use the regex above to handle whitespaces and other quotes.
{
"tailwindCSS.experimental.classRegex": [
// object
// const styles = {
// a: 'bg-black text-ellipsis',
// b: 'text-sm',
// }
["{([\\s\\S]*)}", ":\\s*['\"`]([^'\"`]*)['\"`]"]
]
}
{
"tailwindCSS.experimental.classRegex": [
// object
// const styles = {
// a: 'bg-black text-ellipsis',
// b: 'text-sm',
// }
["{([\\s\\S]*)}", ":\\s*['\"`]([^'\"`]*)['\"`]"]
]
}
Now we have a fully working Tailwind autocomplete for objects!
multiline object
Bonus
A useful trick I learned from this tweet is to turn on editor.quickSuggestions
on strings, so we can trigger suggestions while typing in the string instead of relying on whitespace as the trigger.
"editor.quickSuggestions": {
"strings": "on"
}
"editor.quickSuggestions": {
"strings": "on"
}
string on
string off